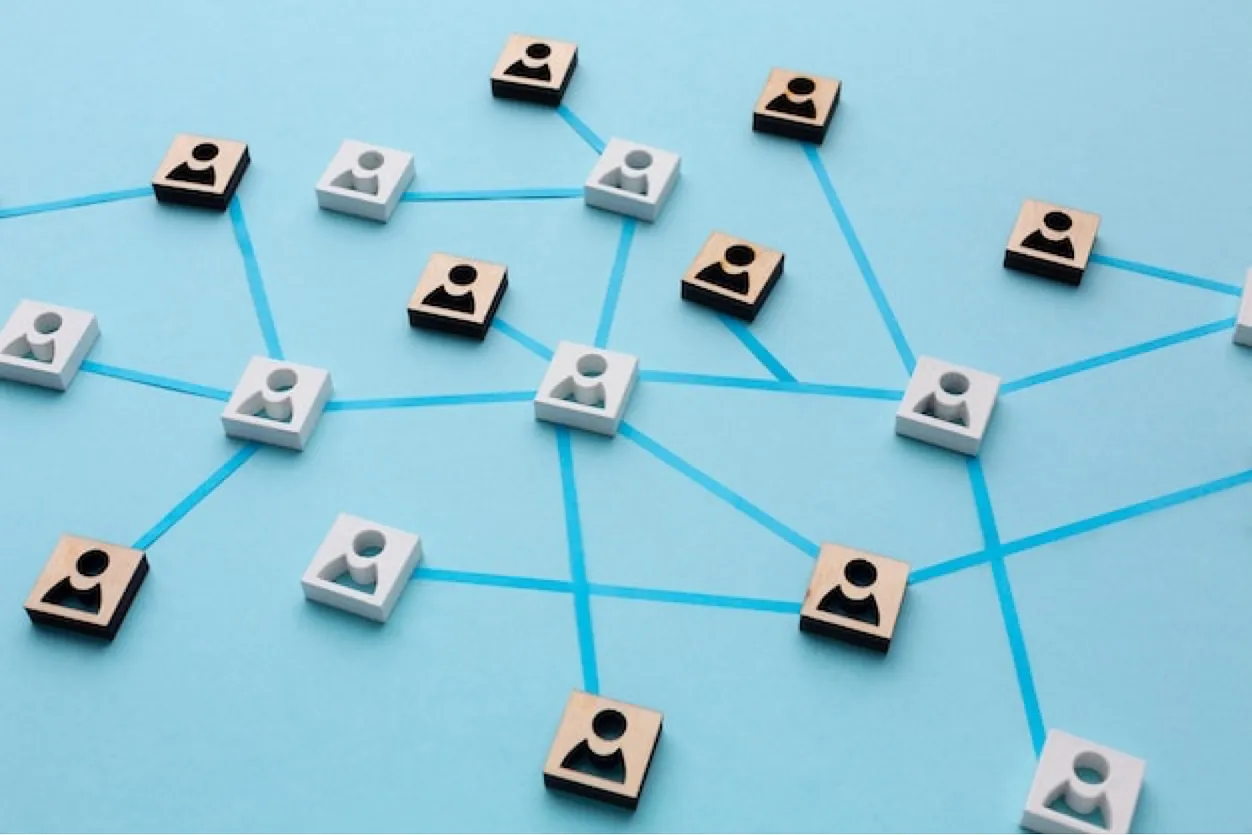
Top 10 Tips to Ace Your Java Concurrency and Multithreading Interview
Halil URAL / August 27, 2024
Are you preparing for a Java Concurrency and Multithreading Interview? If so, you’re in the right place! Interviews focusing on concurrency and multithreading can be quite challenging. But don’t worry – I’m here to help you navigate through it like a pro! Whether you’re a newbie or a seasoned Java developer, this guide will give you all the insights you need to ace your interview. 🌟
Before we dive in, if you find this article helpful, don’t forget to clap, follow, and share! Every little bit of support helps me continue creating valuable content for you. Also, consider supporting me on Ko-Fi to fuel more awesome articles! Support me on Ko-Fi.
1. Understand the Basics of Concurrency and Multithreading 🧠
Before you can master concurrency, you need a strong foundation in the basics. Understand what threads are, how they work, and why they are important in Java. Grasp the concepts of processes vs. threads, multithreading, and the Java Memory Model (JMM).
“You have to learn the rules of the game. And then you have to play better than anyone else.” – Albert Einstein
Java Concurrency is all about multiple threads working simultaneously to complete a task. Multithreading enhances the efficiency of applications by allowing them to perform multiple operations concurrently. Make sure you’re comfortable with how threads are created and managed in Java. Start with the Thread class and Runnable interface, and move up to the Executor framework and ForkJoinPool.
If you want a deep dive into the basics, check out this course: Java Concurrency: The Complete Guide for Beginners.
2. Master Synchronization Techniques 🔐
Synchronization is key to handling shared resources. The concept of synchronization ensures that only one thread can access a resource at a time. Understand the differences between synchronized methods and synchronized blocks. Learn how to use the synchronized keyword effectively to avoid deadlocks and race conditions.
A solid understanding of synchronization also includes mastering locks (ReentrantLock), semaphores, and atomic variables (AtomicInteger, AtomicBoolean, etc.). These tools help you manage threads more efficiently and avoid common pitfalls.
“Success is not the key to happiness. Happiness is the key to success. If you love what you are doing, you will be successful.” – Albert Schweitzer
Synchronized Method
A synchronized method in Java is a method that is used to control access to an object’s state. When a method is declared as synchronized, the thread holds the lock for that object for the duration of the method execution. Other threads cannot enter any synchronized method on the same object until the lock is released.
Here’s an example of a synchronized method:
public class SynchronizedMethodExample {
private int count = 0;
// Synchronized method to increment the count
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
public static void main(String[] args) {
SynchronizedMethodExample example = new SynchronizedMethodExample();
// Creating two threads that call the synchronized method
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
// Starting both threads
thread1.start();
thread2.start();
// Wait for both threads to complete execution
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// Output the final count
System.out.println("Final Count: " + example.getCount());
}
}
Explanation:
- The increment() method is declared as synchronized, ensuring that only one thread can execute it at a time. This prevents multiple threads from updating the count variable simultaneously, which could lead to inconsistent results.
• Two threads (thread1 and thread2) are created, each calling the increment() method 1000 times.
• Because increment() is synchronized, the final count will always be 2000 after both threads complete, demonstrating that the method is thread-safe.
Synchronized Block
A synchronized block is used to synchronize only a part of a method instead of the entire method. It allows for more granular control of synchronization. The block is synchronized on a specific object (also known as the monitor object), and only one thread can execute the code inside this block for a given object monitor.
Here’s an example of a synchronized block:
public class SynchronizedBlockExample {
private int count = 0;
private final Object lock = new Object(); // Custom lock object
// Method containing a synchronized block
public void increment() {
// Only the code inside this block is synchronized
synchronized (lock) {
count++;
}
}
public int getCount() {
return count;
}
public static void main(String[] args) {
SynchronizedBlockExample example = new SynchronizedBlockExample();
// Creating two threads that call the method with a synchronized block
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
// Starting both threads
thread1.start();
thread2.start();
// Wait for both threads to complete execution
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// Output the final count
System.out.println("Final Count: " + example.getCount());
}
}
Explanation:
Instead of synchronizing the entire increment() method, we use a synchronized block to synchronize only the part that increments the count.
• The block is synchronized on the lock object (synchronized (lock)). Only one thread can execute the block at a time for this particular lock object.
• This approach can be more efficient if only a small part of the method needs synchronization, allowing other threads to execute non-synchronized parts concurrently.
Key Differences Between Synchronized Method and Synchronized Block
-
Granularity: A synchronized method locks the entire method, while a synchronized block only locks a specific section of code.
-
Performance: Synchronized blocks can offer better performance by limiting the scope of synchronization, thus reducing contention.
-
Flexibility: Synchronized blocks provide more flexibility since you can synchronize on any object, not just this.
Lock Example
The Lock interface provides a more flexible locking mechanism than the synchronized methods and blocks. One of the most commonly used implementations is ReentrantLock.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private int count = 0;
private final Lock lock = new ReentrantLock(); // Create a ReentrantLock
public void increment() {
lock.lock(); // Acquire the lock
try {
count++; // Critical section
} finally {
lock.unlock(); // Release the lock in the finally block to ensure it's always released
}
}
public int getCount() {
return count;
}
public static void main(String[] args) {
LockExample example = new LockExample();
// Creating two threads that call the increment method
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
// Starting both threads
thread1.start();
thread2.start();
// Wait for both threads to complete execution
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// Output the final count
System.out.println("Final Count: " + example.getCount());
}
}
Example:
• ReentrantLock: This lock allows a thread to re-acquire the same lock if it has already acquired it. It’s called “reentrant” because the lock can be entered multiple times by the same thread without causing a deadlock.
• lock.lock() and lock.unlock(): These methods are used to acquire and release the lock. The lock.unlock() call is placed inside a finally block to ensure that the lock is always released, even if an exception is thrown.
• The program ensures that the count is incremented safely by multiple threads, avoiding race conditions.
Key Differences Between Synchronized Block and Lock
- Use synchronized for simpler synchronization needs, where the capabilities of synchronized are sufficient, and you don’t need advanced features like fair ordering, interruptibility, or multiple condition variables.
• Use Lock (like ReentrantLock) when you need more flexibility and control over locking, need to handle locks in a more fine-grained way, require fairness, need to interrupt threads waiting for locks, or use multiple conditions. However, be mindful of handling lock acquisition and release manually to avoid potential deadlocks or resource leaks.
Semaphore Example
A Semaphore controls access to a resource with a set number of permits. Threads must acquire a permit to access the resource and release it when done.
import java.util.concurrent.Semaphore;
public class SemaphoreExample {
private final Semaphore semaphore = new Semaphore(2); // Only 2 permits available
public void accessResource() {
try {
semaphore.acquire(); // Acquire a permit
System.out.println(Thread.currentThread().getName() + " acquired a permit.");
// Simulate accessing a shared resource
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
System.out.println(Thread.currentThread().getName() + " releasing a permit.");
semaphore.release(); // Release the permit
}
}
public static void main(String[] args) {
SemaphoreExample example = new SemaphoreExample();
// Create multiple threads that attempt to access the resource
for (int i = 0; i < 5; i++) {
new Thread(() -> example.accessResource()).start();
}
}
}
Explanation:
• Semaphore(2): Creates a semaphore with 2 permits, meaning only two threads can access the resource simultaneously.
• semaphore.acquire() and semaphore.release(): acquire() blocks if no permits are available, and release() adds a permit back to the semaphore.
• This example demonstrates controlling concurrent access to a resource. Only two threads can access the resource at a time; other threads must wait until a permit is released.
Atomic Variables Example
Atomic Variables like AtomicInteger, AtomicBoolean, etc., provide a way to update variables in a thread-safe manner without using synchronization. They support atomic operations like incrementAndGet() and compareAndSet().
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicVariableExample {
private final AtomicInteger count = new AtomicInteger(0); // Atomic integer
public void increment() {
count.incrementAndGet(); // Atomic increment
}
public int getCount() {
return count.get();
}
public static void main(String[] args) {
AtomicVariableExample example = new AtomicVariableExample();
// Creating two threads that call the increment method
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
// Starting both threads
thread1.start();
thread2.start();
// Wait for both threads to complete execution
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// Output the final count
System.out.println("Final Count: " + example.getCount());
}
}
Explanation:
• AtomicInteger: Provides atomic operations on integers, ensuring thread-safe updates without using synchronized or lock.
• incrementAndGet(): Atomically increments the current value by 1 and returns the updated value. This operation is atomic, meaning no other thread can see an intermediate value.
• This example shows a simple and efficient way to perform thread-safe operations on shared variables without explicit locking or synchronization.
3. Know the Executor Framework🧵
The Executor framework is an abstraction that provides a mechanism to decouple task submission from the details of how each task will be run, including thread use, scheduling, and more. The ExecutorService interface and its implementations (ThreadPoolExecutor, ScheduledThreadPoolExecutor) are essential for creating and managing a pool of threads efficiently.
Why Use the Executor Framework?
Before diving into the details, let’s understand why the Executor Framework is useful:
-
Decoupling Task Submission from Execution: It separates the submission of tasks from the execution of those tasks. This allows you to focus on the logical work (tasks) you want to perform and not worry about how to manage threads.
-
Thread Pool Management: It provides a mechanism to manage a pool of worker threads, reusing threads instead of creating new ones for each task, which is more efficient and less resource-intensive.
-
Improved Error Handling and Task Scheduling: The framework offers better error handling for tasks that throw exceptions and flexible scheduling capabilities for executing tasks periodically or after a delay.
Core Components of the Executor Framework
The Executor Framework revolves around three key interfaces:
• Executor: The simplest interface that represents an object that executes submitted Runnable tasks.
• ExecutorService: A subinterface of Executor that provides more lifecycle management and task submission capabilities.
• ScheduledExecutorService: An extension of ExecutorService that supports scheduling tasks to run after a delay or periodically.
Example 1: Basic Executor Example
Here’s a basic example of using the Executor interface:
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
public class BasicExecutorExample {
public static void main(String[] args) {
// Create a simple executor using a single thread executor
Executor executor = Executors.newSingleThreadExecutor();
// Define a Runnable task
Runnable task = () -> {
System.out.println("Hello from the Executor Framework!");
};
// Submit the task to the executor
executor.execute(task);
}
}
Explanation: In this example, we create an Executor using Executors.newSingleThreadExecutor(), which creates an executor that uses a single worker thread. We then define a simple task using a Runnable and submit it to the executor.
Example 2: Using ExecutorService with a Thread Pool
The ExecutorService provides more control over task execution and is the preferred way to manage threads.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class ExecutorServiceExample {
public static void main(String[] args) {
// Create a fixed thread pool with 3 threads
ExecutorService executorService = Executors.newFixedThreadPool(3);
// Define multiple Runnable tasks
Runnable task1 = () -> {
System.out.println("Executing Task 1");
};
Runnable task2 = () -> {
System.out.println("Executing Task 2");
};
Runnable task3 = () -> {
System.out.println("Executing Task 3");
};
Runnable task4 = () -> {
System.out.println("Executing Task 4");
};
// Submit tasks to the executor service
executorService.submit(task1);
executorService.submit(task2);
executorService.submit(task3);
executorService.submit(task4);
// Gracefully shut down the executor service
executorService.shutdown();
try {
if (!executorService.awaitTermination(60, TimeUnit.SECONDS)) {
executorService.shutdownNow();
}
} catch (InterruptedException e) {
executorService.shutdownNow();
}
}
}
Explanation:
-
Creating a Fixed Thread Pool: The Executors.newFixedThreadPool(3) creates a thread pool with three threads. This means up to three tasks can be executed concurrently.
-
Submitting Tasks: We submit four tasks to the executor service. The first three tasks will be executed immediately (one per thread), while the fourth will wait until one of the threads becomes free.
-
Shutting Down the Executor Service: After submitting the tasks, we call shutdown() to stop accepting new tasks and start an orderly shutdown of the running tasks. We then use awaitTermination() to wait for the completion of all tasks.
Example 3: ScheduledExecutorService for Scheduled Tasks
ScheduledExecutorService allows you to schedule tasks to run after a delay or periodically.
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledExecutorServiceExample {
public static void main(String[] args) {
// Create a scheduled executor service with a thread pool size of 1
ScheduledExecutorService scheduledExecutorService = Executors.newScheduledThreadPool(1);
// Define a task to run
Runnable task = () -> System.out.println("Running Scheduled Task");
// Schedule the task to run after 5 seconds delay
scheduledExecutorService.schedule(task, 5, TimeUnit.SECONDS);
// Schedule a task to run periodically every 3 seconds
scheduledExecutorService.scheduleAtFixedRate(task, 2, 3, TimeUnit.SECONDS);
// Schedule a task to run with a fixed delay of 4 seconds between the end of one execution and the start of the next
scheduledExecutorService.scheduleWithFixedDelay(task, 2, 4, TimeUnit.SECONDS);
// Optionally, shut down after some time to see periodic tasks in action
try {
Thread.sleep(15000); // let the tasks run for 15 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
scheduledExecutorService.shutdown();
}
}
Explanation:
-
Creating a Scheduled Executor: Executors.newScheduledThreadPool(1) creates a scheduled executor with a single thread.
-
Scheduling Tasks:
• schedule(task, 5, TimeUnit.SECONDS): Schedules the task to run after a delay of 5 seconds.
• scheduleAtFixedRate(task, 2, 3, TimeUnit.SECONDS): Schedules the task to run initially after 2 seconds and then repeatedly every 3 seconds.
• scheduleWithFixedDelay(task, 2, 4, TimeUnit.SECONDS): Schedules the task to run initially after 2 seconds and subsequently with a 4-second delay between the end of one execution and the start of the next.
Example 4: Using Callable and Future
Unlike Runnable, the Callable interface can return a result and throw checked exceptions. Future represents the result of an asynchronous computation.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallableAndFutureExample {
public static void main(String[] args) {
// Create a single thread executor
ExecutorService executorService = Executors.newSingleThreadExecutor();
// Define a Callable task that returns a result
Callable<Integer> task = () -> {
System.out.println("Calculating sum...");
int sum = 0;
for (int i = 0; i <= 10; i++) {
sum = i;
}
return sum;
};
try {
// Submit the task to the executor service
Future<Integer> future = executorService.submit(task);
// Get the result from the future (blocking call)
Integer result = future.get();
System.out.println("Result of the task: " + result);
} catch (Exception e) {
e.printStackTrace();
} finally {
// Shut down the executor service
executorService.shutdown();
}
}
}
Explanation:
-
Defining a Callable Task:
Callable<Integer>
is similar to Runnable but returns a result (Integer in this case) and can throw exceptions. -
Submitting the Task: The task is submitted to the ExecutorService using submit(), which returns a
Future<Integer>
. The Future represents the result of the computation. -
Getting the Result: future.get() is called to block and wait for the result of the computation. If the task completes, the result is printed; if the task throws an exception, it is propagated to the caller.
4. Understand Callable and Future📜
Unlike Runnable, the Callable interface can return a result and throw a checked exception. The Future interface represents the result of an asynchronous computation. It provides methods to check if the computation is complete, wait for its completion, and retrieve the result.
Pro Tip: Prepare for questions on how to handle Future results effectively, including how to use Future.get() without causing your thread to block indefinitely.
Example: Using Callable and Future
Let’s create a simple program that demonstrates the use of Callable and Future. We’ll implement a task that calculates the factorial of a number and returns the result.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallableAndFutureExample {
// Define a Callable class to calculate the factorial of a number
static class FactorialCalculator implements Callable<Integer> {
private final int number;
public FactorialCalculator(int number) {
this.number = number;
}
@Override
public Integer call() throws Exception {
if (number < 0) {
throw new IllegalArgumentException("Number must be non-negative");
}
int factorial= 1;
for (int i= 1; i <= number; i++) {
factorial = i;
// Simulate some delay
Thread.sleep(100);
}
return factorial;
}
}
public static void main(String[] args) {
// Create an ExecutorService with a fixed thread pool of size 2
ExecutorService executorService= Executors.newFixedThreadPool(2);
// Create a Callable task to calculate the factorial of 5
Callable<Integer> task = new FactorialCalculator(5);
// Submit the Callable task to the executor service
Future<Integer> future = executorService.submit(task);
try {
// Perform some other tasks while the factorial is being calculated
System.out.println("Doing some other tasks...");
// Get the result of the future (this will block until the result is available)
Integer result = future.get();
System.out.println("Factorial of 5 is: " + result);
} catch (InterruptedException e) {
System.err.println("Task interrupted");
} catch (ExecutionException e) {
System.err.println("Exception in Callable: " + e.getCause());
} finally {
// Shutdown the executor service
executorService.shutdown();
}
}
}
Explanation:
- FactorialCalculator Class:
• This class implements the Callable<Integer>
interface, meaning it performs a computation that returns an Integer.
• The call() method contains the logic to compute the factorial of a number. It also includes a simulated delay using Thread.sleep(100) to mimic a long-running operation.
• If the input number is negative, it throws an IllegalArgumentException.
- ExecutorService:
• We create an ExecutorService using Executors.newFixedThreadPool(2). This thread pool will manage the execution of our Callable tasks.
- Submitting the Callable Task:
• The FactorialCalculator is submitted to the executor service using the submit() method, which returns a Future<Integer>
. This Future represents the result of the computation that will be available once the task is complete.
- Getting the Result from Future:
• The future.get() method is called to retrieve the result of the computation. If the computation isn’t finished yet, this call will block until the result is ready.
• If the task completes normally, get() returns the result; if the task throws an exception, get() throws an ExecutionException, which wraps the original exception thrown by the Callable.
- Handling Exceptions:
• InterruptedException is thrown if the current thread is interrupted while waiting for the result.
• ExecutionException is thrown if the call() method of the Callable throws an exception. We use e.getCause() to get the actual exception thrown.
- Shutting Down the Executor Service:
• Finally, we shut down the executor service to free up resources. This is a good practice to prevent resource leaks.
5. Be Ready for Deadlocks and Thread Safety Questions 🕵️♂️
Interviewers often test your understanding of deadlocks and how to avoid them. A deadlock occurs when two or more threads are blocked forever, waiting for each other. Be prepared to discuss deadlock prevention techniques such as:
• Lock ordering: Always acquire locks in a fixed order.
• Lock timeout: Use lock timeout to detect deadlocks.
• Deadlock detection: Implement a deadlock detection algorithm.
Thread safety is another crucial topic. Make sure you know how to make classes immutable and the best practices to make your code thread-safe.
Example of Lock Ordering
Consider a scenario where we have two locks, lock1 and lock2, and two threads, Thread A and Thread B. To prevent deadlocks, we should ensure that both threads acquire the locks in the same order.
Here’s a small code snippet that demonstrates lock ordering:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockOrderingExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void method1() {
// Always acquire lock1 first, then lock2
lock1.lock();
try {
System.out.println("Lock 1 acquired by " + Thread.currentThread().getName());
lock2.lock();
try {
System.out.println("Lock 2 acquired by " + Thread.currentThread().getName());
// Critical section that requires both locks
System.out.println("Executing critical section in method1 by " + Thread.currentThread().getName());
} finally {
lock2.unlock();
System.out.println("Lock 2 released by " + Thread.currentThread().getName());
}
} finally {
lock1.unlock();
System.out.println("Lock 1 released by " + Thread.currentThread().getName());
}
}
public void method2() {
// Always acquire lock1 first, then lock2
lock1.lock();
try {
System.out.println("Lock 1 acquired by " + Thread.currentThread().getName());
lock2.lock();
try {
System.out.println("Lock 2 acquired by " + Thread.currentThread().getName());
// Critical section that requires both locks
System.out.println("Executing critical section in method2 by " + Thread.currentThread().getName());
} finally {
lock2.unlock();
System.out.println("Lock 2 released by " + Thread.currentThread().getName());
}
} finally {
lock1.unlock();
System.out.println("Lock 1 released by " + Thread.currentThread().getName());
}
}
public static void main(String[] args) {
LockOrderingExample example = new LockOrderingExample();
// Create two threads that call the two methods
Thread thread1 = new Thread(example::method1, "Thread 1");
Thread thread2 = new Thread(example::method2, "Thread 2");
// Start both threads
thread1.start();
thread2.start();
}
}
Explanation:
- Two Locks (lock1 and lock2):
• We have two locks represented by ReentrantLock instances (lock1 and lock2).
- Method Locking Order:
• In both method1() and method2(), the locks are acquired in the same order: lock1 first, then lock2.
• This consistent lock ordering across methods prevents a potential deadlock. Even if Thread 1 and Thread 2 try to execute their respective methods simultaneously, they will never get into a state where one thread holds lock1 and the other holds lock2, both waiting indefinitely for the other lock.
- Avoiding Deadlock with Lock Ordering:
• By always acquiring lock1 before lock2, we ensure a consistent locking order. This prevents the cyclic dependency that causes deadlocks.
• If Thread 1 starts and acquires lock1 first, Thread 2 will wait until lock1 is released before acquiring it, thus preventing deadlock.
- Output:
• The console output will show that both threads acquire and release the locks in a consistent order without any deadlock.
Example of Lock Timeout
Here’s a small code snippet demonstrating the use of tryLock() with a timeout:
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockTimeoutExample {
private final Lock lock = new ReentrantLock();
public void performTask() {
boolean lockAcquired = false;
try {
// Try to acquire the lock within 2 seconds
lockAcquired = lock.tryLock(2, TimeUnit.SECONDS);
if (lockAcquired) {
// Critical section
System.out.println(Thread.currentThread().getName() + " acquired the lock and is performing the task.");
// Simulate some work with the lock held
Thread.sleep(1000);
} else {
System.out.println(Thread.currentThread().getName() + " could not acquire the lock within the timeout.");
}
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
if (lockAcquired) {
lock.unlock();
System.out.println(Thread.currentThread().getName() + " released the lock.");
}
}
}
public static void main(String[] args) {
LockTimeoutExample example = new LockTimeoutExample();
Runnable task = example::performTask;
// Create two threads that try to perform the task
Thread thread1 = new Thread(task, "Thread 1");
Thread thread2 = new Thread(task, "Thread 2");
// Start both threads
thread1.start();
thread2.start();
}
}
Explanation:
- ReentrantLock:
• We create a ReentrantLock instance called lock to control access to the shared resource.
- tryLock(long timeout, TimeUnit unit):
• The tryLock() method with a timeout is used to attempt to acquire the lock. It tries to acquire the lock for up to 2 seconds (TimeUnit.SECONDS).
• If the lock is acquired within the timeout, the method returns true; otherwise, it returns false.
- Handling Lock Acquisition:
• If the lock is acquired successfully, the thread enters the critical section, simulates some work with Thread.sleep(1000), and then releases the lock.
• If the lock is not acquired within the timeout, the thread prints a message indicating it couldn’t acquire the lock.
- Thread Behavior:
• Two threads (Thread 1 and Thread 2) attempt to execute the performTask() method concurrently.
• If Thread 1 acquires the lock first, Thread 2 will attempt to acquire the lock for 2 seconds. If it cannot acquire the lock within that time, it prints a message and exits the method.
6. Get Comfortable with volatile Keyword ⚡
The volatile keyword in Java is used to indicate that a variable’s value will be modified by different threads. Declaring a variable as volatile ensures that its value is read from the main memory, and not from the thread’s local cache.
This concept is often misunderstood, so make sure you understand when and how to use volatile correctly.
When to Use volatile
• Use volatile when you want to ensure that all threads see the most recent write to a variable.
• It is useful for flags and state-check variables that are shared between threads.
• It is not a replacement for synchronization; it only ensures visibility and not atomicity. For example, operations like i++ are not atomic even if i is declared volatile.
Example of Using volatile
Here’s a simple example that demonstrates how the volatile keyword ensures visibility of shared variables across threads.
public class VolatileExample {
// Declare a volatile boolean flag
private volatile boolean running = true;
public void startTask() {
System.out.println("Task started...");
// Run the task in a separate thread
new Thread(() -> {
while (running) { // Loop runs while running is true
// Simulate some work with a sleep
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Task stopped.");
}).start();
}
public void stopTask() {
System.out.println("Stopping task...");
running = false; // Set the flag to false to stop the task
}
public static void main(String[] args) {
VolatileExample example = new VolatileExample();
// Start the task in a separate thread
example.startTask();
// Main thread sleeps for a while
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Stop the task
example.stopTask();
}
}
Explanation:
- Volatile Variable:
• The variable running is declared volatile. This means that every time a thread reads running, it gets the latest value from the main memory, and every time it writes to running, the updated value is immediately visible to other threads.
- Start Task:
• The startTask() method starts a new thread that continuously checks the value of the running variable in a while loop. As long as running is true, the loop continues, simulating some ongoing task with a sleep.
- Stop Task:
• The stopTask() method sets running to false. Since running is volatile, the change is immediately visible to the thread running the task. The next time the loop checks the running variable, it reads false from the main memory and exits the loop.
- Main Thread Control:
• In the main() method, we start the task and let it run for a short while (500 milliseconds). Then, we call stopTask() to stop the running task.
Why volatile is Needed in This Example
Without the volatile keyword, the running variable may be cached locally in the thread running the loop. This means the loop might not see the updated value (false) immediately, and the program could potentially run indefinitely. The volatile keyword ensures that the most recent value of running is always read from the main memory, so the loop will exit promptly when stopTask() is called.
7. Learn About Java Memory Model (JMM) and Happens-Before Relationship 🏗️
The Java Memory Model (JMM) defines how threads interact through memory and what behaviors are allowed in concurrent execution. The happens-before relationship guarantees that memory writes by one specific statement are visible to another specific statement.
Understanding the JMM is crucial for writing correct concurrent programs. Make sure you are familiar with terms like visibility, ordering, and atomicity.
8. Practice Common Concurrency Problems 🔄
Practice makes perfect! Focus on common concurrency problems such as:
• Producer-Consumer Problem: Understand how to use BlockingQueue.
• Dining Philosophers Problem: Learn about deadlock handling.
• Read-Write Locking: Use ReentrantReadWriteLock for scenarios with frequent reads and infrequent writes.
9. Familiarize Yourself with Java Concurrency Utilities 🛠️
Java provides a wide array of concurrency utilities like CountDownLatch, CyclicBarrier, Exchanger, and Phaser. Each of these utilities has its own use case and knowing when to use which can make or break your interview.
For a deeper understanding of these utilities, read: Core Java Concurrency
10. Java Concurrency Collections 💥
Java Concurrency Collections are part of the java.util.concurrent package, introduced in Java 5 to provide thread-safe, efficient alternatives to the standard collections framework. These collections are designed to handle concurrent access and modification by multiple threads without requiring explicit synchronization.
Why Use Concurrency Collections?
-
Thread Safety: Concurrency collections are inherently thread-safe, meaning they can be safely accessed and modified by multiple threads simultaneously.
-
Performance: These collections are optimized for concurrent operations, often using lock-free algorithms or fine-grained locking to minimize contention and improve performance.
-
Ease of Use: They simplify concurrent programming by providing ready-to-use, thread-safe collection implementations that don’t require manual synchronization.
Key Concurrency Collection Classes
Some of the most commonly used concurrency collections in Java include:
-
ConcurrentHashMap: A thread-safe version of HashMap that allows concurrent read and write operations without locking the entire map.
-
CopyOnWriteArrayList: A thread-safe version of ArrayList where all mutative operations (like add and remove) are implemented by making a fresh copy of the underlying array.
-
ConcurrentLinkedQueue: A thread-safe, non-blocking, unbounded queue based on linked nodes. It uses a lock-free algorithm for better performance in concurrent environments.
-
BlockingQueue (e.g., ArrayBlockingQueue, LinkedBlockingQueue, PriorityBlockingQueue): These queues support operations that wait for the queue to become non-empty when retrieving an element and for space to become available in the queue when storing an element.
-
ConcurrentSkipListMap and ConcurrentSkipListSet: Thread-safe, sorted collections based on skip lists, offering scalable performance for concurrent access.
Example: Using ConcurrentHashMap
Let’s take a look at a simple example that demonstrates the use of ConcurrentHashMap, a thread-safe map that supports full concurrency of retrievals and adjustable expected concurrency for updates.
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class ConcurrentHashMapExample {
// Create a ConcurrentHashMap to store word counts
private static ConcurrentHashMap<String, Integer> wordCountMap = new ConcurrentHashMap<>();
public static void main(String[] args) {
// Sample text
String text = "the quick brown fox jumps over the lazy dog the fox is quick and the dog is lazy";
// Split the text into words
String[] words = text.split(" ");
// Create a fixed thread pool with 4 threads
ExecutorService executorService = Executors.newFixedThreadPool(4);
// Submit tasks to the executor service for counting words
for (String word : words) {
executorService.submit(() -> countWord(word));
}
// Shutdown the executor service and wait for tasks to complete
executorService.shutdown();
try {
executorService.awaitTermination(1, TimeUnit.MINUTES);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Print the final word counts
System.out.println("Word counts: " + wordCountMap);
}
// Method to count the occurrences of a word in a thread-safe manner
private static void countWord(String word) {
wordCountMap.merge(word, 1, Integer::sum);
}
}
Final Thoughts
Java Concurrency and Multithreading can be challenging topics, but with the right preparation, you can master them. Remember to build a strong foundation, practice regularly, and stay updated with the latest Java developments.
“The secret of getting ahead is getting started.” – Mark Twain
If you found this guide helpful, don’t forget to clap, follow, and share! Also, consider subscribing to my Medium channel for more in-depth articles like this: Subscribe Here. And if you’d like to support my work, you can buy me a coffee on Ko-Fi! Support me on Ko-Fi.
Good Luck Guyzzz! 😊